Are you ready to ace your next Angular interview? In a world where web development is the backbone of modern business, Angular stands out as a powerhouse framework that developers can't afford to overlook. Whether you're a seasoned pro or just starting out, understanding Angular is crucial for anyone aiming to make their mark in the industry. But how do you prepare for those tough interview questions that separate the skilled from the truly proficient?
The right preparation can be the difference between standing out or blending in with the crowd. In this guide, we’ll dive into the most critical Angular interview questions for 2024, ensuring you walk into that interview room with confidence and the knowledge to impress.
Ace your front-end interview with our 2024 cheat sheet! Learn the ins and outs of HTML, CSS, and JavaScript for guaranteed success.
Core Angular Concepts

Understanding the core concepts of Angular is essential for any developer aiming to excel in this framework. Let’s dive into some of the foundational elements:
- Components: The building blocks of an Angular application, components control a patch of screen called a view. Each component consists of a TypeScript class, an HTML template, and CSS styles.
- Modules: Angular apps are modular, meaning they are split into functional blocks called modules. Each module can contain components, services, and other code.
- Services and Dependency Injection: Services in Angular are used for sharing data and logic across components. Dependency Injection (DI) is a design pattern Angular uses to efficiently manage these services.
- Directives: Angular directives are special instructions in the DOM. There are three types: component, structural, and attribute directives.
- Routing: Angular's Router module allows you to create and manage navigation between views, ensuring a seamless user experience.
- Data Binding: Angular supports one-way and two-way data binding, enabling efficient communication between the view and the component.
- Pipes: Pipes transform data in the template before displaying it, allowing for cleaner and more readable code.
- Lifecycle Hooks: Angular components go through several phases, from creation to destruction, which are managed by lifecycle hooks like ngOnInit, ngOnDestroy, etc.
Don't miss out on your dream full-stack job in 2024! Our full-stack interview guide will equip you with the knowledge you need to succeed.
Most Asked Angular Interview Questions

To help you ace your next Angular interview, here’s a curated list of 40 questions ranging from basic to advanced, each followed by a thorough explanation.
1. What is Angular, and how does it differ from AngularJS?
Angular is a platform and framework for building single-page client applications using HTML and TypeScript. It differs from AngularJS, which was the original version of the framework, in terms of architecture (component-based vs. MVC), language (TypeScript vs. JavaScript), and performance optimizations, among other aspects.
2. Explain the role of a component in Angular.
A component in Angular controls a portion of the UI through a class (handles logic), a template (handles view), and styles. Components are reusable and can be nested to build complex UIs.
3. What is a module in Angular?
A module is a container for a coherent block of code dedicated to an application domain, workflow, or closely related set of capabilities. Angular applications are modular and typically start with a root module, AppModule, which bootstraps the application.
4. Describe Dependency Injection in Angular.
Dependency Injection (DI) is a design pattern used to implement IoC (Inversion of Control). Angular uses DI to provide components with their dependencies without the component needing to create them manually. This makes the code more modular and easier to test.
5. How does Angular handle routing?
Angular uses the Router module to define navigation paths within the application. Routes are defined in a configuration, mapping URLs to components. This allows for navigation between different views or pages within the application without reloading the page.
6. What are Angular Directives?
Directives in Angular are used to manipulate the DOM. Structural directives (like *ngIf and *ngFor) alter the layout by adding or removing elements, while attribute directives (like ngClass and ngStyle) modify the appearance or behavior of an element.
7. What is data binding in Angular, and what types are there?
Data binding in Angular allows communication between the component and the DOM. There are two types:
- One-way binding: Data flows from the component to the template.
- Two-way binding: Data flows in both directions, allowing the view to reflect changes in the component and vice versa. This is typically done using ngModel.
8. Explain Angular's Lifecycle Hooks.
Lifecycle hooks are special methods in Angular that allow you to tap into key moments in a component’s lifecycle:
ngOnInit(): Called once, after the first ngOnChanges().
ngOnChanges(): Called whenever an input property changes.
ngOnDestroy(): Called just before the component is destroyed.
ngDoCheck(): Detects and acts upon changes that Angular doesn't catch on its own.
9. What are Angular Pipes?
Answer: Pipes are used to transform data in the template. Angular provides several built-in pipes, such as DatePipe, UpperCasePipe, LowerCasePipe, and CurrencyPipe. You can also create custom pipes for more complex transformations.
10. How does Angular handle forms?
Angular provides two approaches to handling forms:
- Template-driven forms: Forms are defined in the template with directives to bind to data.
- Reactive forms: Forms are defined in the component class with more explicit control over the data and validation.
11. What is Angular CLI, and how does it help in development?
Angular CLI is a command-line interface that automates tasks like creating projects, adding files, running tests, and deploying. It provides a consistent development environment and follows best practices.
12. How do you create a service in Angular?
Answer: Services in Angular are created using the @Injectable decorator and can be provided in a module or component. They are typically used to encapsulate business logic, data access, and communication with external services.
13. Explain Angular’s change detection mechanism.
Angular’s change detection checks for changes in the component's state and updates the DOM accordingly. Angular uses zone.js to track asynchronous operations and mark components for check.
14. What are the differences between ngIf and ngSwitch?
- ngIf removes or recreates a portion of the DOM tree based on an expression.
- ngSwitch is similar but allows for multiple mutually exclusive elements to be created or removed based on the expression value.
15. What is Angular’s AOT compilation?
Ahead-of-Time (AOT) compilation is the process of compiling Angular HTML and TypeScript code into efficient JavaScript code during the build phase before the browser downloads and runs the code. This improves performance and ensures that the application is fully compiled when it loads.
16. How do you optimize an Angular application?
Optimizing an Angular application involves several strategies:
- Use AOT compilation.
- Implement lazy loading for modules.
- Optimize change detection with OnPush strategy.
- Minimize the bundle size using tree-shaking.
- Implement caching and service workers.
17. What is the role of Angular Universal?
Angular Universal is a technology that allows you to render Angular applications on the server. This results in faster initial load times and better SEO, as the content is already available when the page is loaded.
18. Explain the difference between Observable and Promise in Angular.
- Observable: Supports multiple values over time, allows cancellation, and supports operators for complex operations.
- Promise: Handles a single future value or error and is simpler but less flexible.
19. How do you manage state in Angular applications?
State management in Angular can be handled using services, or more advanced libraries like NgRx, which is based on Redux and provides a way to manage application state in a predictable manner.
20. What are Angular Animations, and how do you implement them?
Angular animations are a powerful way to create visual effects in your application. They can be implemented using the Angular @angular/animations package, which provides a set of tools to define complex animations with trigger, state, and transition functions.
21. What is Lazy Loading in Angular, and why is it important?
Lazy Loading is a design pattern that loads modules as needed rather than all at once. This improves the initial load time and overall performance by reducing the size of the initial bundle.
22. How do you handle errors in Angular applications?
Error handling in Angular can be done using try-catch blocks, Angular’s HttpClient interceptors for handling HTTP errors, and global error handling using the ErrorHandler class.
23. What is NgZone in Angular?
NgZone is a service provided by Angular to help manage change detection in asynchronous tasks. It allows developers to run code outside of the Angular zone to avoid triggering unnecessary change detection cycles.
24. Explain the use of the trackBy function in Angular.
The trackBy function is used in Angular's *ngFor directive to optimize rendering by identifying items in a collection uniquely. By default, Angular re-renders the entire list if any item changes. Using trackBy, you can track each item by a unique identifier, like an ID, so Angular only re-renders the items that have changed, improving performance.
25. What are Angular decorators, and name some commonly used ones?
Decorators in Angular are functions that add metadata to classes, methods, or properties, allowing Angular to understand how to process them. Some commonly used decorators include:
- @Component: Defines a component.
- @NgModule: Declares a module.
- @Injectable: Marks a class as available to be injected.
- @Input: Allows a parent component to bind to a child component's property.
- @Output: Allows a child component to emit events to a parent component.
26. What is NgRx in Angular, and how does it handle state management?
NgRx is a state management library for Angular based on Redux. It provides a way to manage the application’s state in a predictable manner. NgRx uses actions to describe state changes, reducers to handle state transitions, selectors to query the state, and effects to handle side effects like HTTP requests. This structure ensures that the state is immutable and that the changes are predictable, making the application easier to debug and test.
Action -> Reducer -> New State -> Component -> Effect (for side effects)
27. Explain how Angular's Change Detection works, and how you can optimize it.
Angular’s Change Detection mechanism checks for changes in the application’s model and updates the view accordingly. It runs after every asynchronous event like HTTP requests, timers, or user interactions. By default, Angular uses the default Change Detection strategy, which checks all components. You can optimize this using the OnPush Change Detection strategy, which only checks a component when its input properties change or when an event is emitted from the component itself. This reduces the number of checks and improves performance, especially in large applications.
Event -> Change Detection Cycle -> Component Tree Check -> DOM Update (if changes are found)
27. Explain how Angular's Change Detection works, and how you can optimize it.
Angular’s Change Detection mechanism checks for changes in the application’s model and updates the view accordingly. It runs after every asynchronous event like HTTP requests, timers, or user interactions. By default, Angular uses the default Change Detection strategy, which checks all components. You can optimize this using the OnPush Change Detection strategy, which only checks a component when its input properties change or when an event is emitted from the component itself. This reduces the number of checks and improves performance, especially in large applications.
Change Detection Process:
Event -> Change Detection Cycle -> Component Tree Check -> DOM Update (if changes are found)
28. What are Angular Modules, and how do you implement Lazy Loading with them?
Angular Modules are used to group related code together, such as components, services, directives, and pipes, into cohesive blocks. Lazy Loading is a technique used to load Angular modules only when they are needed, which improves the application’s startup time by reducing the initial bundle size.
To implement Lazy Loading, you define routes in your AppRoutingModule that load modules asynchronously using the loadChildren property.
For example:
Event -> Change Detection Cycle -> Component Tree Check -> DOM Update (if changes are found)
28. What are Angular Modules, and how do you implement Lazy Loading with them?
Angular Modules are used to group related code together, such as components, services, directives, and pipes, into cohesive blocks. Lazy Loading is a technique used to load Angular modules only when they are needed, which improves the application’s startup time by reducing the initial bundle size.
To implement Lazy Loading, you define routes in your AppRoutingModule that load modules asynchronously using the loadChildren property. For example:

30. Explain how Angular's Renderer2 differs from direct DOM manipulation.
Renderer2 is a service provided by Angular to perform DOM manipulations in a platform-agnostic way. Direct DOM manipulation using methods like document.querySelector can break Angular’s rendering model and make the application less portable, especially in environments like server-side rendering (Angular Universal) or native mobile apps (NativeScript). Renderer2 provides methods like createElement, setStyle, and appendChild to safely interact with the DOM, ensuring that the application remains consistent across different platforms.
31. How does Angular handle Security, and what practices should be followed to ensure a secure application?
Angular has several built-in security features to protect applications from common vulnerabilities such as Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and Clickjacking. Angular automatically sanitizes potentially dangerous content in templates, and it provides mechanisms to handle authentication and authorization securely.
Security Best Practices:
- Always use Angular’s built-in sanitization and escape mechanisms.
- Avoid using the innerHTML directive unless absolutely necessary, and sanitize the content first.
- Implement proper authentication and authorization using libraries like OAuth2 or JWT.
- Regularly update Angular and third-party libraries to avoid known vulnerabilities.
32. Describe the difference between ViewChild and ContentChild in Angular.
ViewChild: It is used to query and interact with child components, directives, or DOM elements that are part of the component's template. It’s typically used to access elements and components that are within the same view.
ContentChild: It is used to query and interact with projected content, i.e., elements or components that are passed into the component using <ng-content> slots.

What is the difference between Angular’s Template-Driven and Reactive Forms?
- Template-Driven Forms: These forms are simpler to implement and are defined directly in the template using Angular’s directives like ngModel. They are suitable for simple forms but offer less control over the form’s behavior and validation.
- Reactive Forms: These forms are more complex but offer greater control over the form’s data model. They are defined programmatically in the component class using Angular’s FormGroup, FormControl, and FormArray classes. Reactive forms are ideal for complex forms with dynamic validation and complex interaction.
34. How does Angular's HttpClient handle Interceptors, and what are common use cases?
Interceptors in Angular are used to inspect and transform HTTP requests and responses globally. By implementing HttpInterceptor, you can modify requests before they are sent to the server, handle responses before they reach the application, and perform actions like adding authentication tokens, logging, or error handling.
Common Use Cases:
- Adding Authorization Headers: Attach JWT tokens or API keys to every request.
- Logging: Record the details of every request and response for debugging.
- Global Error Handling: Catch HTTP errors across the application and display a user-friendly message.
Interceptor example:

35. Explain Angular's Dependency Injection Hierarchical System.
Angular’s Dependency Injection (DI) system is hierarchical, meaning that services can be provided at different levels in the application, and the injector will follow the hierarchy to resolve dependencies. There are three main levels:
- Root Level: Services provided in AppModule or with the providedIn: 'root' option are singleton and available throughout the application.
- Module Level: Services provided in a specific module are singleton within that module and shared across components within the module.
- Component Level: Services provided at the component level are available only to that component and its children, ensuring encapsulation.
This hierarchy allows for flexibility and fine-grained control over service lifetimes and scope.
36. What is Angular’s Dynamic Component Loader, and how is it used?
Angular’s Dynamic Component Loader allows you to create and insert components into the DOM at runtime. This is useful for scenarios where the components to be displayed are not known at compile-time, such as rendering dynamic content based on user actions or configuration data.
37. How does Angular handle multi-environment configuration?
Angular provides a built-in way to manage different configurations for different environments (e.g., development, staging, production). This is achieved using the angular.json configuration file, which allows you to define environment-specific settings and file replacements.
Steps to Set Up Multi-Environment Configuration:
- Define environment files like environment.ts, environment.prod.ts.
- Specify file replacements in angular.json.
- Access environment variables using import { environment } from 'src/environments/environment';.
38. What is Angular’s Ivy Renderer, and how does it improve the framework?
Ivy is the new rendering engine introduced in Angular 9, replacing the previous View Engine. Ivy improves the framework by making it faster, smaller, and more flexible. It enables faster compilation, better tree-shaking (eliminating unused code), and more straightforward debugging. Additionally, Ivy makes Angular applications more backward-compatible and future-proof, ensuring that even large applications can benefit from performance optimizations without requiring extensive changes.
39. Explain the role of Angular Schematics and how they can be customized.
Angular Schematics are templates used by the Angular CLI to generate boilerplate code for common tasks like creating components, services, modules, and more. Schematics can be customized or created from scratch to fit specific project needs, ensuring that the code generated follows the project’s conventions and best practices.
Example of Custom Schematic:
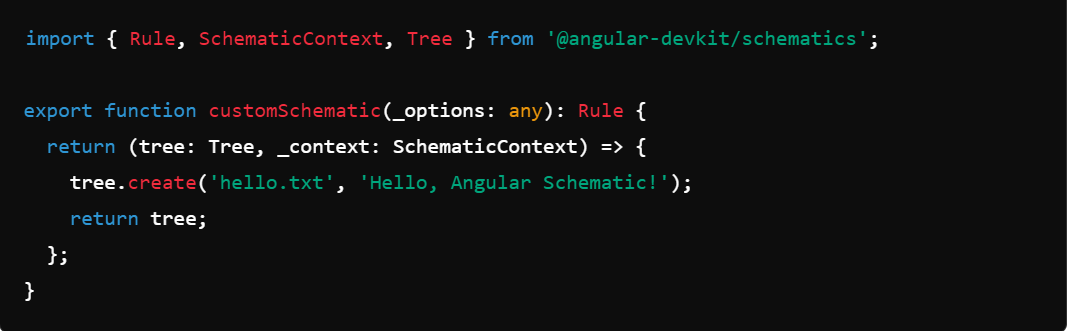
40. How do you implement Role-Based Access Control (RBAC) in an Angular application?
Role-Based Access Control (RBAC) in Angular can be implemented using route guards and directives. Route guards like CanActivate and CanLoad can be used to prevent unauthorized users from accessing specific routes. Directives can be used to conditionally display elements based on the user’s roles.
Example of RBAC Implementation:

Conquer your web developer interview fears with our guide that offers essential questions, coding tips, and strategies to help you ace your next interview.
Conclusion
Mastering these Angular interview questions will give you a solid foundation to tackle any challenges that come your way during your job hunt in 2024. But remember, landing a top engineering role isn't just about knowing the right answers—it's also about connecting with the right opportunities.
If you're a company looking to hire top-tier engineers or a candidate seeking your next big opportunity, consider using Weekday. Weekday helps companies hire engineers vouched for by other techies, with a sourcing engine that operates on auto-pilot. Within just four days of signing up, you’ll start receiving candidates with ready back-channel references, making your hiring process smoother and more efficient. Backed by Y-Combinator and ranked #1 on Product Hunt, Weekday is your go-to platform for hiring engineers who are truly a cut above the rest.
Ready to elevate your hiring process? Check out Weekday.works today!