Preparing for an ASP.NET interview can be daunting, but having a solid grasp of the key concepts and common questions can make all the difference. This guide aims to provide you with the most up-to-date and relevant ASP.NET interview questions and answers for 2024, helping you to confidently showcase your expertise.
In this guide, we'll cover a range of topics from the basics of ASP.NET Core to more advanced concepts such as middleware, dependency injection, and routing. Whether you're just starting or looking to refresh your knowledge, these questions and answers will help you understand what interviewers are looking for and how to articulate your responses effectively.
As you go through these questions, you'll gain insights into the practical applications of ASP.NET, best practices for development, and the latest features that are essential for modern web development. This preparation will not only help you answer questions accurately but also demonstrate your problem-solving skills and depth of understanding.
Let’s dive in and equip you with the knowledge and confidence needed to excel in your next ASP.NET interview!
Preparation Tips
Expected Foundational Knowledge
To ace your ASP.NET interview, it's crucial to have a strong foundation in several core areas:
- C#: Ensure you are comfortable with C#, the primary programming language used in ASP.NET development. Familiarize yourself with syntax, data types, control structures, and exception handling. Understand advanced topics like LINQ, asynchronous programming, and delegates.
- Object-Oriented Programming (OOP): Master the principles of OOP, such as inheritance, polymorphism, encapsulation, and abstraction. Be ready to discuss how these principles are applied in ASP.NET.
- HTML, CSS, and JavaScript: A good grasp of front-end technologies is essential. HTML and CSS are fundamental for structuring and styling web pages, while JavaScript is crucial for creating interactive and dynamic user experiences.
Overview of ASP.NET MVC and Web API Frameworks
Understanding the frameworks used in ASP.NET development is key to impressing your interviewers:
- ASP.NET MVC (Model-View-Controller): This framework is designed for building web applications that follow the MVC pattern.
- Model: Represents the application's data and business logic.
- View: Handles the display and presentation of data.
- Controller: Manages user input and updates the Model and View accordingly.
- Be prepared to explain how MVC promotes separation of concerns, improves testability, and supports clean, maintainable code.
- ASP.NET Web API: This framework is ideal for building HTTP services that can be consumed by various clients, including browsers and mobile devices.
- RESTful Services: Understand the principles of REST and how Web API facilitates the creation of RESTful services.
- HTTP Methods: Be familiar with GET, POST, PUT, DELETE, and PATCH, and how they correspond to CRUD operations.
- Routing: Know how routing works in Web API, including attribute routing and route templates.
Interactive Tips for Mastery
- Build Sample Projects: Apply what you've learned by building small projects. This hands-on experience is invaluable and helps reinforce your understanding.
- Join Online Communities: Engage with ASP.NET communities on forums, GitHub, and social media. Discussing problems and solutions with peers can provide new insights and help you stay updated.
- Practice Mock Interviews: Simulate the interview experience with a friend or mentor. Practice answering questions out loud to improve your communication skills and confidence.
- Use Online Resources: Leverage online tutorials, documentation, and courses to deepen your knowledge. Websites like Microsoft Learn and Pluralsight offer excellent materials for all skill levels.
By mastering these foundational areas and understanding the core frameworks of ASP.NET, you'll be well-prepared to confidently and competently tackle your interview.
Basic ASP.NET Interview Questions

What is ASP.NET?
ASP.NET is a powerful framework developed by Microsoft for building dynamic web applications and services. It allows developers to create enterprise-class web applications with minimal coding by providing a unified programming model and necessary services. ASP.NET is built on the Common Language Runtime (CLR), which means developers can write ASP.NET code using any supported .NET language, such as C# or VB.NET. It supports various development styles, including server-side, client-side, and hybrid applications, making it a versatile choice for web development.
What are the different types of ASP.NET applications?
ASP.NET supports several types of applications to cater to different development needs:
- Web Forms: A traditional web application model that allows for event-driven development. It uses a drag-and-drop, WYSIWYG (What You See Is What You Get) designer and is well-suited for rapid development.
- MVC (Model-View-Controller): A design pattern that promotes separation of concerns, making the application more modular and easier to manage. MVC provides better control over HTML, CSS, and JavaScript.
- Web API: A framework for building HTTP services that can be consumed by various clients, including browsers, mobile devices, and tablets. It is ideal for creating RESTful services.
- Blazor: A framework for building interactive web UIs using C# instead of JavaScript. Blazor allows for the development of client-side applications with WebAssembly and server-side applications.
What is the difference between ASP and ASP.NET?
ASP (Active Server Pages):
- Technology: Script-based technology that uses interpreted scripts.
- Language: Primarily uses VBScript for server-side scripting.
- Performance: Generally slower due to interpreted scripts.
- Features: Limited support for object-oriented programming and modern development practices.
ASP.NET:
- Technology: Compiled, event-driven technology that uses the .NET framework.
- Language: Supports multiple languages, including C#, VB.NET, and more.
- Performance: Faster execution due to compiled code and efficient caching mechanisms.
- Features: Rich object-oriented features, supports code-behind model, includes robust server controls and services, and integrates with modern development tools and practices.
What are the advantages of ASP.NET?
- Performance: Compiled code, just-in-time compilation, and caching mechanisms improve performance significantly.
- Security: Built-in Windows authentication, request validation, and membership providers enhance security.
- Scalability and Simplicity: The framework is designed for easy scalability and maintainability, making it suitable for both small and large applications.
- Rich Toolbox and Designer: Visual Studio provides a rich toolbox with drag-and-drop server controls, WYSIWYG editing, and debugging tools.
- Cross-Platform: ASP.NET Core enables cross-platform development, allowing applications to run on Windows, macOS, and Linux.
- Robust Framework: Comprehensive libraries and support for modern web development practices, including RESTful APIs, WebSockets, and asynchronous programming.
What is the ASP.NET page life cycle?
The ASP.NET page life cycle consists of several stages, each playing a crucial role in processing a web request:
- Page Request: ASP.NET determines whether the request is for a cached page or requires processing.
- Start Initialization of page properties, such as Request and Response.
- Initialization: Controls on the page are initialized and assigned a unique ID.
- Load: The page and its controls are loaded with data. Control properties are set to their default values.
- Postback Event Handling: Handles events triggered by user actions, such as button clicks.
- Rendering: The page and its controls are rendered into HTML to be sent to the client.
- Unload: Cleanup code is executed, such as closing database connections and releasing resources.
What is the view state in ASP.NET?
View State is a mechanism for preserving the state of web controls between postbacks. It stores the values of controls in a hidden field on the page, allowing the page to remember user inputs and control states across postbacks. View State is encoded and sent to the client as part of the page, ensuring that the state is maintained without requiring server-side storage. This feature simplifies the development of interactive web applications by reducing the need to manually handle state management.
What is the difference between Server.Transfer and Response.Redirect?
Server.Transfer:
- Method: Transfers the request to another page on the server without making a round-trip back to the client's browser.
- URL: The URL in the browser does not change, making the transfer transparent to the user.
- Performance: More efficient as it avoids an additional HTTP request.
- Use Case: Suitable for server-side redirects where the URL does not need to be exposed to the client.
Response.Redirect:
- Method: Sends an HTTP response to the client's browser, instructing it to request a different URL.
- URL: The URL in the browser changes to the new address.
- Performance: Less efficient due to the additional round-trip HTTP request.
- Use Case: Suitable for client-side redirects where the user needs to see the new URL.
What is the difference between Session and Application state in ASP.NET?
Session State:
- Scope: Maintains per-user session state.
- Lifetime: Lasts for the duration of a user's session, typically until the user logs out or the session times out.
- Storage: Stored in memory, database, or state server.
- Usage: Used to store user-specific data, such as login credentials, user preferences, and shopping cart contents.
Application State:
- Scope: Shared across all users and sessions.
- Lifetime: Lasts for the lifetime of the application, from start to shutdown.
- Storage: Stored in memory on the server.
- Usage: Used to store global data, such as application-wide settings, configuration information, and counters.
What is the purpose of the web.config file?
The web.config file is a configuration file used in ASP.NET web applications. It is an XML file that contains configuration settings and information specific to the application. Key purposes include:
- Security Configurations: Manage authentication, authorization, and custom error pages.
- Application Settings: Store application-specific settings and connection strings.
- Session State Management: Configure session state modes and timeout settings.
- Custom Error Handling: Define custom error pages for handling exceptions.
- URL Rewriting: Configure URL rewriting rules for better SEO and user-friendly URLs.
- Assembly References: Manage and reference external assemblies.
What is the difference between ASP.NET Web Forms and ASP.NET MVC?
ASP.NET Web Forms:
- Architecture: Uses an event-driven development model with a rich set of server controls.
- State Management: Uses view state to maintain control state across postbacks.
- Development Style: Drag-and-drop development with Visual Studio designer support.
- Use Case: Suitable for rapid development and applications with less complex UI interactions, where ease of development is a priority.
ASP.NET MVC:
- Architecture: Follows the Model-View-Controller pattern, promoting separation of concerns.
- State Management: Does not use view state, allowing for better control over HTML output and page performance.
- Development Style: Full control over HTML, CSS, and JavaScript, making it easier to create clean and maintainable code.
- Use Case: Suitable for complex applications requiring fine-grained control over behavior, scalability, and testability.
What is the purpose of the Global.asax file?
The Global.asax file, also known as the ASP.NET application file, contains event handlers for application-level events. It allows developers to write code that responds to application-wide events such as:
- Application Start: Code to run when the application starts, typically used for initialization tasks.
- Application End: Code to run when the application ends, used for cleanup tasks.
- Session Start: Code to run when a new user session starts.
- Session End: Code to run when a user session ends.
- Application Error: Code to handle unhandled exceptions globally.
The Global.asax file provides a central place to manage these events, making it easier to implement application-wide logic and handle global application events.
What is bundling and minification in ASP.NET?
Bundling: The process of combining multiple CSS or JavaScript files into a single file. This reduces the number of HTTP requests needed to load a web page, improving load times and overall performance.
Minification: The process of removing unnecessary characters (such as whitespace, comments, and line breaks) from CSS or JavaScript files. This reduces the file size, further improving load times and performance.
Together, bundling and minification help optimize web applications by reducing the number of requests and the size of files that need to be downloaded, leading to faster page load times and a better user experience. ASP.NET includes built-in support for these techniques, making it easy to implement them in your applications.
ASP.NET Intermediate Interview Questions
ASP.NET Core's Support for Caching to Enhance Performance
ASP.NET Core provides robust caching mechanisms to enhance application performance by reducing response times and server load. The primary caching strategies include:
- In-Memory Caching: Stores data in the server's memory, allowing for extremely fast access. This is ideal for frequently accessed data that doesn't change often, such as configuration settings.
- Distributed Caching: Useful for applications deployed across multiple servers. It stores data in a distributed cache, such as Redis or SQL Server, enabling shared access across the entire server farm.
- Response Caching: Caches HTTP responses on the server side to serve repeated requests faster. This reduces the need to process the same request multiple times.
- Output Caching: Similar to response caching but allows caching of specific parts of the response, improving efficiency in scenarios where only parts of the page change.
These caching mechanisms significantly improve application performance by reducing database queries, minimizing processing time, and enhancing user experience through faster load times.
Explain the Importance of MVC in ASP.NET Core
The Model-View-Controller (MVC) design pattern is fundamental to ASP.NET Core for several key reasons:
- Separation of Concerns: MVC divides an application into three main components:
- Model: Represents the application's data and business logic.
- View: Manages the display and presentation of data.
- Controller: Handles user input and interactions, updating the Model and View accordingly. This separation makes the application more modular and easier to manage.
- Maintainability: By keeping the application's logic, UI, and user interactions separate, MVC simplifies debugging, testing, and updating parts of the application without affecting others.
- Flexibility: MVC allows for more flexible and testable code structures. Developers can work on different components (Model, View, Controller) simultaneously without conflicts.
- Reusability: Components in MVC can be reused across different parts of the application or even in different projects, enhancing productivity and reducing redundancy.
Describe the Roles of .csproj, NuGet, Program Class, Startup Class, and wwwroot in ASP.NET Core
.csproj (C# Project File): An XML-based file that defines the project’s configuration, including target frameworks, package dependencies, and build settings. It ensures that the project is correctly built and its dependencies are managed efficiently.
NuGet Package Manager: A tool integrated into Visual Studio that manages project dependencies. It allows developers to easily install, update, and manage third-party libraries and tools from the NuGet repository, ensuring that all necessary packages are available for the project to compile and run.
Program Class: The entry point of an ASP.NET Core application. It configures and runs the web host, setting up the server and starting the application's request-processing pipeline. The CreateHostBuilder method configures the host with defaults and services.
Startup Class: Defines the configuration for the application's services and the middleware pipeline. It includes:
- ConfigureServices Method: Registers services required by the application.
- Configure Method: Defines how the application responds to HTTP requests by setting up the middleware pipeline.
wwwroot Folder: The default folder for serving static files such as HTML, CSS, JavaScript, and images. This folder is accessible publicly, allowing these files to be directly requested by clients, improving the efficiency of serving static content.
How Does ASP.NET Core Utilize Caching for Performance Enhancement?
ASP.NET Core utilizes caching to enhance performance through several mechanisms:
- In-Memory Caching: Stores frequently accessed data in the server's memory for rapid retrieval, reducing the need to repeatedly fetch data from the database.
- Distributed Caching: Uses external caching solutions like Redis or SQL Server to store data across multiple servers. This is beneficial for load-balanced environments where data consistency across servers is crucial.
- Response Caching: Caches HTTP responses to quickly serve repeated requests, reducing server processing time and database load.
- Output Caching: Caches parts of the web page output, which is useful when only certain sections of a page change frequently. This reduces the overhead of generating the entire page repeatedly.
By implementing these caching strategies, ASP.NET Core applications can achieve faster response times, reduce server load, and provide a smoother user experience.
Discuss the Concept of Middleware in ASP.NET Core
Middleware in ASP.NET Core is a fundamental concept for handling HTTP requests and responses. Each middleware component is a piece of software that processes requests as they move through the pipeline. Key characteristics and roles of middleware include:
- Request Processing: Middleware components can inspect, modify, or terminate requests before they reach the next component.
- Response Processing: They can also inspect or modify the response before it is sent back to the client.
- Modularity: Middleware components are added to the pipeline in the Startup.Configure method, allowing developers to customize the request-processing pipeline.
- Common Middleware: Examples include authentication, logging, exception handling, static file serving, and URL rewriting.
Middleware components are executed in the order they are added to the pipeline, providing a flexible and modular approach to handling HTTP requests and responses.
Explain Setting Up Entity Framework Core in ASP.NET Core
Entity Framework Core (EF Core) is a modern object-database mapper for .NET. Setting up EF Core in an ASP.NET Core application involves several steps:
Install EF Core Packages: Add the necessary EF Core packages via NuGet. For example:

Create the DbContext Class: Define a class that inherits from ‘DbContext’, which represents a session with the database and provides methods for querying and saving data.
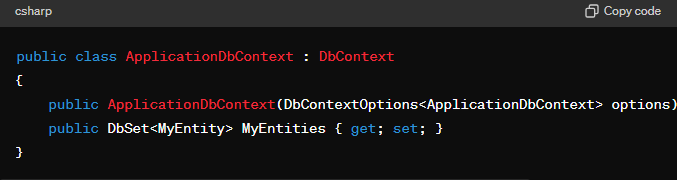
Configure the DbContext: Register the ‘DbContext’ in the ‘ConfigureServices’ method of the Startup class

Add Connection String: Define the connection string in the appsettings.json file.

Run Migrations: Use EF Core tools to create and apply database migrations.

These steps configure EF Core in the ASP.NET Core application, enabling it to manage database operations effectively.
How Does ASP.NET Core Integrate with Client-Side Frameworks?
ASP.NET Core integrates seamlessly with client-side frameworks, enhancing the development of rich, interactive web applications. Integration methods include:
- Single Page Applications (SPAs): ASP.NET Core can serve as the backend for SPAs built with frameworks like Angular, React, and Vue.js. Tools like the ASP.NET Core SPA templates simplify this integration.
- Tag Helpers: Provide server-side processing of HTML elements, enabling easier integration with client-side frameworks.
- Razor Pages and Components: Allow for the development of dynamic web pages with minimal JavaScript. Blazor, a feature of ASP.NET Core, enables C# to be used in client-side development.
- API Controllers: ASP.NET Core Web API can serve RESTful endpoints that client-side frameworks consume.
What Are the Benefits of Using Razor Pages in ASP.NET Core?
Razor Pages is a page-based programming model in ASP.NET Core that offers several benefits:
- Simplicity: Provides a straightforward way to build web applications by organizing code with a single-page model.
- Separation of Concerns: Keeps the code-behind logic separate from the HTML, improving maintainability.
- Productivity: Reduces boilerplate code and simplifies the development process, allowing for rapid development.
- Enhanced Functionality: Supports features like dependency injection, model binding, and tag helpers, enhancing the development experience.
Describe the Purpose of Tag Helpers in ASP.NET Core
Tag Helpers in ASP.NET Core are server-side components that help in generating dynamic HTML content. They provide a way to:
- Improve HTML Syntax: Enable server-side code to participate in creating and rendering HTML elements.
- Simplify Razor Syntax: Make Razor markup more readable and maintainable.
- Enhance Productivity: Reduce the amount of boilerplate code needed to perform common tasks, such as form input validation and URL generation.
Tag Helpers enhances the development experience by combining the power of server-side logic with the simplicity of HTML.
Explain the Roles of .csproj, NuGet, Program Class, Startup Class, and wwwroot in ASP.NET Core
- .csproj (C# Project File):
- Manages the configuration and dependencies of the project.
- Specifies the target framework, build settings, and included packages.
- Critical for compiling and building the application correctly.
- NuGet Package Manager:
- Handles the installation, update, and management of third-party libraries and tools.
- Integrates seamlessly with Visual Studio, simplifying the addition of dependencies.
- Ensures that all required packages are available and up-to-date.
- Program Class:
- The entry point of the application, contains the Main method.
- Configures and starts the web host, setting up the server to handle incoming requests.
- Typically sets up logging and configuration sources.
- Startup Class:
- Defines the services and middleware pipeline for the application.
- ConfigureServices method: Registers services like MVC, EF Core, and custom services.
- Configure method: Sets up the middleware components that process HTTP requests.
- wwwroot Folder:
- The designated folder for serving static files such as HTML, CSS, JavaScript, and images.
- Publicly accessible and directly served to clients.
- Helps in organizing and managing static assets efficiently.
How Does ASP.NET Core Utilize Caching for Performance Enhancement?
ASP.NET Core employs several caching strategies to boost performance:
- In-Memory Caching:
- Stores frequently accessed data in server memory for fast retrieval.
- Ideal for small to medium-sized data that doesn’t change frequently.
- Distributed Caching:
- Uses external caching systems like Redis or SQL Server.
- Shares cached data across multiple servers, ensuring consistency and scalability in distributed environments.
- Response Caching:
- Caches HTTP responses to serve repeated requests quickly.
- Reduces server workload by avoiding repeated processing for the same request.
- Output Caching:
- Caches specific parts of the response, improving efficiency for partial page updates.
- Useful for scenarios where only certain sections of a page change frequently.
These caching mechanisms help reduce latency, lower server load and provide a better user experience by delivering faster responses.
Discuss the Concept of Middleware in ASP.NET Core
Middleware in ASP.NET Core is a core concept that involves processing HTTP requests and responses in a modular pipeline. Each middleware component can perform specific tasks, such as:
- Authentication: Verifying user credentials.
- Authorization: Ensuring the user has permission to access resources.
- Logging: Recording details about requests and responses.
- Static File Serving: Providing static files like images, CSS, and JavaScript.
- Error Handling: Managing exceptions and generating appropriate responses.
Middleware components are added to the request pipeline in the Startup.Configure method. They are executed in the order they are added, allowing developers to build flexible and modular request-processing pipelines.
Explain Setting Up Entity Framework Core in ASP.NET Core
Setting up Entity Framework Core (EF Core) in an ASP.NET Core application involves these steps:
1. Install EF Core Packages:
Add necessary packages via NuGet, such as Microsoft.EntityFrameworkCore and Microsoft.EntityFrameworkCore.SqlServer.
2. Create the DbContext Class:
- Define a class that inherits from DbContext, representing a session with the database.
- Configure DbSet properties for each entity.

3. Configure the DbContext:
- Register the DbContext in the ConfigureServices method of the Startup class.
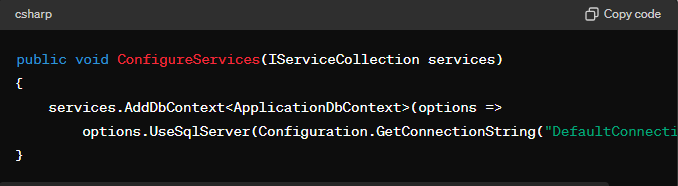
4. Add Connection String:
- Define the connection string in the appsettings.json file.

5. Run Migrations:
- Use EF Core tools to create and apply database migrations.

These steps configure EF Core, enabling database operations and data management within the ASP.NET Core application.
ASP.NET Core Advanced Interview Questions
Q. Deep Dive into Model Binding in ASP.NET Core
Model binding in ASP.NET Core is the process of creating .NET objects from HTTP requests. This allows developers to work with strongly-typed objects rather than raw request data, simplifying the handling of user inputs and making code more maintainable.
Key Features:
- Simple and Complex Types: Model binding supports both simple types (e.g., integers, strings) and complex types (e.g., custom objects with multiple properties). It automatically converts data from HTTP requests to the appropriate .NET types.
- Binding Sources: Data can come from various sources such as form fields, query strings, route data, and request bodies. Attributes like [FromQuery], [FromRoute], [FromForm], and [FromBody] specify the binding source explicitly.
- Validation: Integrated with the validation framework, model binding helps ensure that data is valid before it is processed further. Invalid data can be automatically flagged, and appropriate error messages can be generated.
Example Explanation:
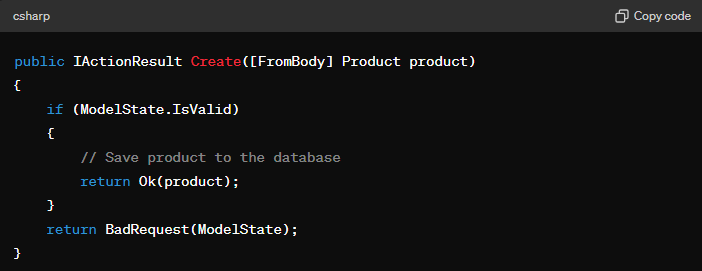
In this example, the Create action method takes a Product object from the request body. The model binder automatically converts the JSON in the request body to a Product instance. The ModelState.IsValid check ensures that the data is valid according to the model's validation rules before proceeding.
Action Method Characteristics and Usage
Action methods in ASP.NET Core are endpoints that handle HTTP requests. They are defined within controllers and execute the application's business logic.
Key Characteristics:
- Return Types: Action methods can return various types, including IActionResult, specific result types (e.g., OkResult, BadRequestResult), or custom objects that are serialized to JSON or XML.
- HTTP Methods: Action methods are mapped to specific HTTP verbs using attributes like [HttpGet], [HttpPost], [HttpPut], and [HttpDelete].
- Parameter Binding: Parameters can be automatically bound from different parts of the request (e.g., URL, query string, request body) using model binding.
Example Explanation:

This GetProduct method handles GET requests to retrieve a product by its ID. The {id} in the route template specifies that the method expects an ID parameter from the URL. The method returns an ‘Ok’ result with the product if found, or a NotFound result if the product does not exist.
Explaining the IActionResult Interface and Its Implementations
The IActionResult interface defines a contract for action results in ASP.NET Core. It provides a standard way to handle HTTP responses in a controller action.
Key Implementations:
- ContentResult: Returns a string as the response body.
- JsonResult: Returns JSON-formatted data.
- FileResult: Returns file content, useful for file downloads.
- StatusCodeResult: Returns a specific HTTP status code.
- ViewResult: Renders a view as HTML.
- RedirectResult: Redirects to another URL.
Example Explanation:
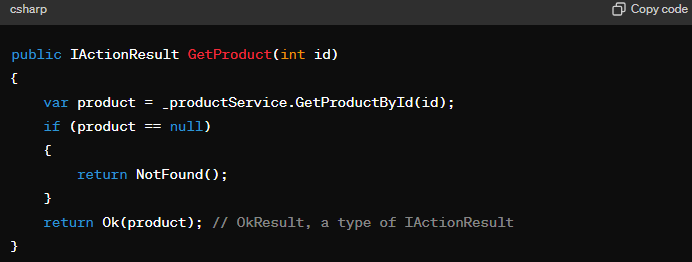
Dependency Injection in ASP.NET Core: Principles and Implementation
Dependency Injection (DI) in ASP.NET Core is a design pattern used to achieve Inversion of Control (IoC) between classes and their dependencies. It makes applications more modular, testable, and maintainable.
Principles:
- Inversion of Control: The framework, rather than the application, controls the creation and lifecycle of dependencies.
- Service Lifetime: Services can be registered with different lifetimes:
- Transient: Created each time they are requested.
- Scoped: Created once per request.
- Singleton: Created once and shared throughout the application's lifetime.
- Service Registration: Dependencies are registered in the Startup.ConfigureServices method.
Implementation Explanation:
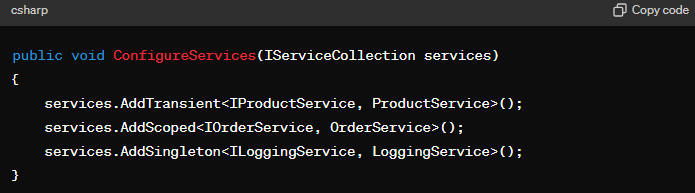
In this example, three services are registered with different lifetimes. IProductService is registered as transient, meaning a new instance is created each time it is requested. IOrderService is scoped, meaning it is created once per request. ILoggingService is singleton, meaning a single instance is created and shared throughout the application's lifetime.
Middleware: Configuration and Role in ASP.NET Core Applications
Middleware in ASP.NET Core processes requests and responses in a pipeline. Each middleware component can perform operations on the HTTP context before passing it to the next component.
Configuration:
- Adding Middleware: Middleware is added in the Startup.Configure method using the IApplicationBuilder interface.
- Order of Execution: Middleware components are executed in the order they are added.
Role:Middleware components handle various tasks such as authentication, logging, error handling, serving static files, and URL rewriting.
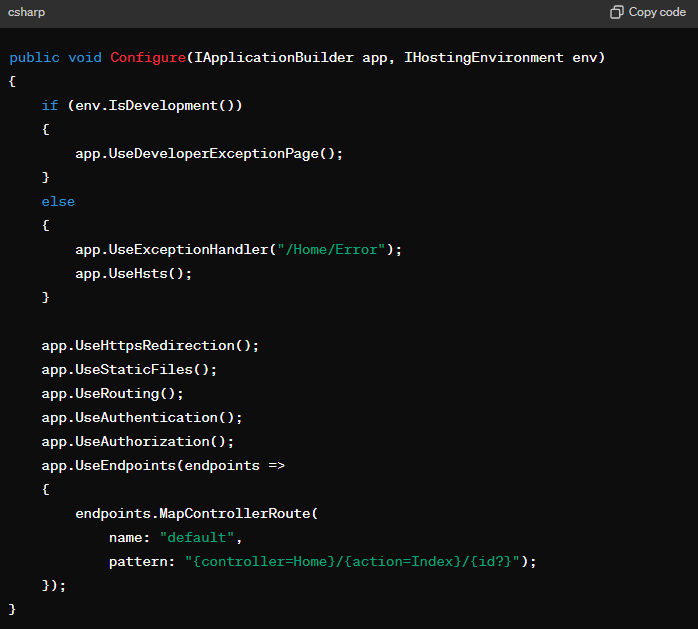
In this example, three services are registered with different lifetimes. IProductService is registered as transient, meaning a new instance is created each time it is requested. IOrderService is scoped, meaning it is created once per request. ILoggingService is singleton, meaning a single instance is created and shared throughout the application's lifetime.
Middleware: Configuration and Role in ASP.NET Core Applications
Middleware in ASP.NET Core processes requests and responses in a pipeline. Each middleware component can perform operations on the HTTP context before passing it to the next component.
Configuration:
- Adding Middleware: Middleware is added in the Startup.Configure the method using the IApplicationBuilder interface.
- Order of Execution: Middleware components are executed in the order they are added.
Role: Middleware components handle various tasks such as authentication, logging, error handling, serving static files, and URL rewriting.
Utilizing Entity Framework for Object-Relational Mapping
Entity Framework (EF) Core is an ORM (Object-Relational Mapper) for .NET, enabling developers to interact with databases using .NET objects.
Key Features:
- Code-First Approach: Developers define the database schema using C# classes, and EF Core generates the database.
- Database-First Approach: EF Core can generate C# classes from an existing database schema.
- LINQ Queries: Allows querying the database using LINQ, providing a powerful and type-safe way to interact with data.
Example Explanation:

In this example, ApplicationDbContext is a DbContext class representing a session with the database. It includes a DbSet property for the Product entity. The ConfigureServices method registers ApplicationDbContext with the dependency injection container and configures it to use a SQL Server database with a connection string defined in appsettings.json.
Understanding HTTP Context in Controllers
The HttpContext object in ASP.NET Core encapsulates all HTTP-specific information about an individual HTTP request.
Key Properties and Methods:
- Request: Contains details about the incoming HTTP request, such as headers, query strings, and form data.
- Response: Used to configure the HTTP response, including setting status codes and writing response body content.
- Session: Provides access to session data, allowing for state management across requests.
- User: Represents the authenticated user making the request, including claims and roles.
Usage Example Explanation:

In this example, the GetUserAgent action method accesses the HttpContext.Request.Headers collection to retrieve the User-Agent header, which provides information about the client making the request. The Content method is used to return the user agent string as the response body.
Explain Custom Model Binders in ASP.NET Core
Custom model binders in ASP.NET Core allow developers to handle complex binding scenarios that the default model binder cannot. They provide flexibility for binding special data types or handling custom data transformations.
Steps to Create a Custom Model Binder:
- Implement IModelBinder: Define a class that implements the IModelBinder interface and provides logic for binding the model.
Explanation:

This custom model binder parses a date string from the request and binds it to a DateTime object. If parsing fails, it sets the binding result to failed.
- Create a ModelBinderProvider: Define a provider that registers the custom model binder.
Explanation:
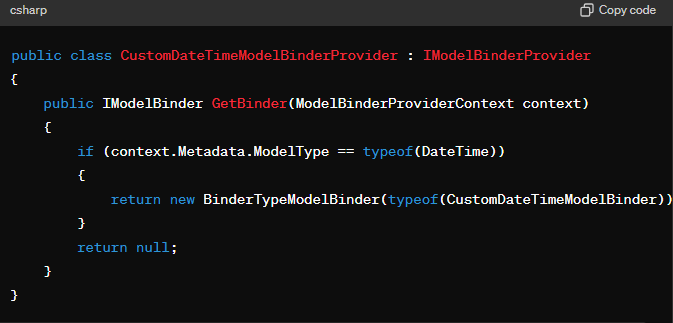
This provider checks if the model type is DateTime and returns the custom model binder if it is.
- Register the Model Binder: Register the custom model binder provider in the Startup.ConfigureServices method.
Explanation:
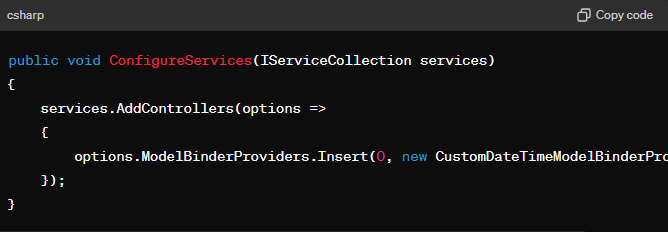
This code registers the custom model binder provider, ensuring that the custom binder is used whenever a DateTime parameter is encountered.
Custom model binders provide a powerful way to handle complex data binding scenarios, making ASP.NET Core applications more flexible and robust.
Explain Custom Model Binders in ASP.NET Core
Custom model binders in ASP.NET Core allow developers to create bespoke logic for binding complex types or transforming data from HTTP requests into .NET objects. This is particularly useful when the default model binder cannot handle certain scenarios or when specific formatting or validation is required.
Steps to Create a Custom Model Binder:
- Implement IModelBinder:
- Create a class that implements the IModelBinder interface and provides custom logic for binding.
Example and Explanation:

This example defines a custom binder that parses a date string from the request and binds it to a DateTime object. If parsing fails, it sets the binding result to failed.
2. Create a ModelBinderProvider:
- Define a provider that registers the custom model binder.
Example and Explanation:
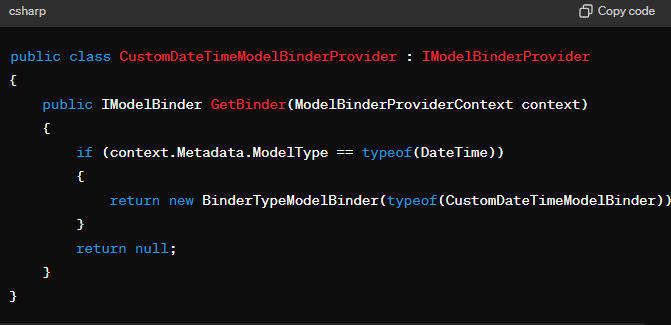
This provider checks if the model type is DateTime and returns the custom model binder if it is.
3. Register the Model Binder:
- Register the custom model binder provider in the Startup.ConfigureServices method.
Example and Explanation:

This code registers the custom model binder provider, ensuring the custom binder is used for DateTime parameters.
Custom model binders enhance flexibility, allowing for tailored data processing and binding scenarios in ASP.NET Core applications.
Discuss Action Method Characteristics in ASP.NET Core Controllers
Action methods in ASP.NET Core controllers are the core endpoints that handle HTTP requests. These methods are responsible for processing incoming requests, executing business logic, and returning responses to the client.
Key Characteristics:
- Return Types: Action methods can return various types:
- IActionResult: Provides a flexible way to return different types of HTTP responses.
- JsonResult, ContentResult, ViewResult: Specific result types for JSON data, plain text, and rendered views, respectively.
- Custom Objects: Automatically serialized to JSON or XML.
- Attributes: Attributes like [HttpGet], [HttpPost], [HttpPut], and [HttpDelete] specify the HTTP method the action handles.
- Parameter Binding: Action methods can have parameters bound from the request using model binding attributes like [FromQuery], [FromRoute], [FromForm], and [FromBody].
Example and Explanation:
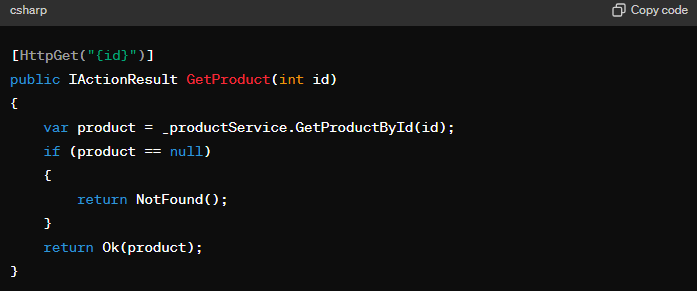
Describe the IActionResult Interface and Its Implementations
The IActionResult interface defines a contract for action results in ASP.NET Core, providing a standard way to handle HTTP responses in controller actions.
Key Implementations:
- ContentResult: Returns a string as the response body.
- JsonResult: Returns JSON-formatted data.
- FileResult: Returns file content, suitable for file downloads.
- StatusCodeResult: Returns a specific HTTP status code.
- ViewResult: Renders a view as HTML.
- RedirectResult: Redirects to another URL.
Example and Explanation:

In this example, GetProduct returns an OkResult with the product if it exists, or a NotFoundResult if the product is not found. Both OkResult and NotFoundResult are implementations of IActionResult, allowing for flexible response handling.
Discuss Dependency Injection Principles and Implementation in ASP.NET Core
Dependency Injection (DI) in ASP.NET Core is a design pattern that promotes the separation of concerns by decoupling object creation from business logic. This makes applications more modular, testable, and maintainable.
Principles:
- Inversion of Control: The framework, rather than the application, controls the creation and lifecycle of dependencies.
- Service Lifetime:
- Transient: Created each time they are requested.
- Scoped: Created once per request.
- Singleton: Created once and shared throughout the application's lifetime.
- Service Registration: Dependencies are registered in the Startup.ConfigureServices method.
Implementation Explanation:
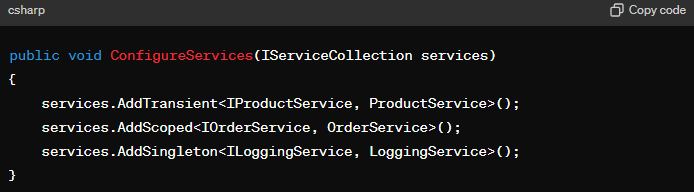
In this example, services are registered with different lifetimes. IProductService is transient, IOrderService is scoped, and ILoggingService is singleton. This ensures the correct lifecycle for each service based on its usage requirements.
Explain Middleware Configuration in ASP.NET Core
Middleware in ASP.NET Core processes requests and responses in a pipeline. Each middleware component can perform operations on the HTTP context before passing it to the next component.
Configuration:
- Adding Middleware: Middleware is added in the Startup.Configure the method using the IApplicationBuilder interface.
- Order of Execution: Middleware components are executed in the order they are added.
Role: Middleware components handle various tasks such as authentication, logging, error handling, serving static files, and URL rewriting.
Example and Explanation:
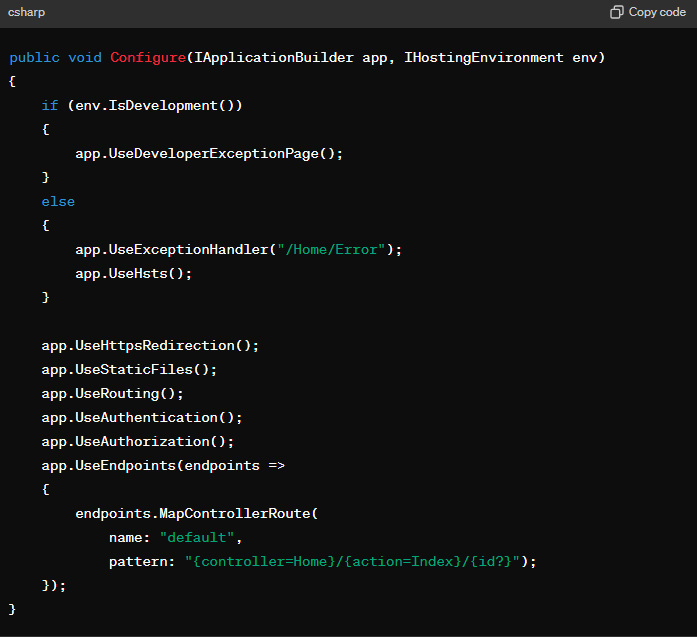
In this example, middleware components are configured to handle requests and responses. The pipeline includes exception handling, static file serving, routing, authentication, and authorization.
Explain Accessing HttpContext in ASP.NET Core Controllers
The HttpContext object in ASP.NET Core encapsulates all HTTP-specific information about an individual HTTP request.
Key Properties and Methods:
- Request: Contains details about the incoming HTTP request, such as headers, query strings, and form data.
- Response: Used to configure the HTTP response, including setting status codes and writing response body content.
- Session: Provides access to session data, allowing for state management across requests.
- User: Represents the authenticated user making the request, including claims and roles.
Usage Example and Explanation:

In this example, the GetUserAgent action method accesses the ‘HttpContext.Request.Headers’ collection to retrieve the User-Agent header, which provides information about the client making the request. The Content method is used to return the user agent string as the response body. This demonstrates how HttpContext can be utilized to access request-specific information within a controller.
Deployment and Best Practices
1. The Deployment Process for an ASP.NET Core Application
Deploying an ASP.NET Core application involves several steps to ensure the application is correctly configured, built, and hosted. The process can vary slightly depending on the hosting environment, such as Windows with IIS, Linux with Nginx, or cloud platforms like Azure.
Steps for Deployment:
- Preparation:
- Ensure the project is correctly configured with necessary dependencies, configurations, and environment variables.
- Set the appropriate environment (Development, Staging, Production) in the launchSettings.json file or through environment variables.
- Build and Publish:
- Use the dotnet publish command to compile the application and prepare it for deployment. This command creates a self-contained or framework-dependent deployment package.
- Set Up the Hosting Environment:
- Windows with IIS: Install the .NET Core Hosting Bundle, which includes the ASP.NET Core Module for IIS. Configure IIS to point to the published folder and set up the application pool.
- Linux with Nginx: Install .NET Core Runtime, configure Nginx as a reverse proxy, and set up a systemd service to manage the application.
- Cloud Platforms: Configure the cloud environment (e.g., Azure App Service) to deploy the application from a repository or upload the published package.
- Deploy and Test:
- Copy the published files to the server or deploy them via a CI/CD pipeline.
- Verify the deployment by accessing the application and checking for any issues or errors.
Example for IIS Deployment:
1. Publish the Application:

2. Configure IIS:
- Install the .NET Core Hosting Bundle.
- Create a new website in IIS and point it to the ./publish folder.
- Configure the application pool to use the correct .NET Core version.
2. Internet Information Services (IIS) and Kestrel Web Server: Configuration and Differences
IIS (Internet Information Services):
- Configuration:
- Install the .NET Core Hosting Bundle.
- Use IIS Manager to create and configure websites.
- Configure application pools and bindings.
- Use the ASP.NET Core Module to proxy requests to the Kestrel server.
- Pros:
- Rich set of features for hosting, security, and management.
- Integrated with Windows Authentication and other Windows services.
- Supports multiple applications and sites on a single server.
- Cons:
- More complex configuration compared to Kestrel alone.
- Overhead due to additional layers.
Kestrel Web Server:
- Configuration:
- Kestrel is the default web server included with ASP.NET Core.
- Configure Kestrel in the Program.cs file using the CreateHostBuilder method.
- Use middleware and configuration files to manage settings.
- Pros:
- Lightweight and fast, ideal for microservices and containers.
- Supports HTTPS and other essential web features.
- Can be used as a standalone server or behind a reverse proxy like Nginx or IIS.
- Cons:
- Fewer built-in features compared to IIS.
- Requires additional configuration for production scenarios (e.g., reverse proxy setup).
Example Kestrel Configuration:
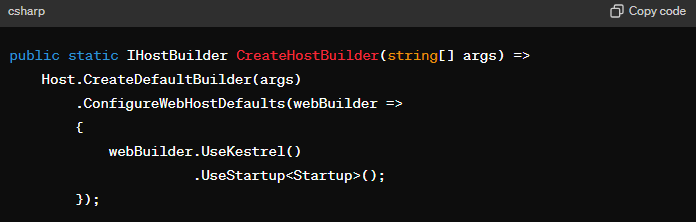
3. Best Practices for Writing Efficient, Maintainable ASP.NET Core Applications
1. Code Organization:
- Use a clear and consistent project structure.
- Follow SOLID principles to ensure modularity and maintainability.
- Separate concerns by organizing code into layers (e.g., Data, Business, Presentation).
2. Dependency Injection:
- Leverage the built-in DI container to manage dependencies.
- Register services with appropriate lifetimes (Transient, Scoped, Singleton).
3. Configuration Management:
- Use appsettings.json for configuration settings and environment-specific files (e.g., appsettings.Development.json).
- Store sensitive information in environment variables or secret management tools.
4. Logging and Monitoring:
- Implement structured logging using built-in logging providers (e.g., Serilog, NLog).
- Monitor application performance and health using tools like Application Insights.
5. Security Practices:
- Use HTTPS for all communication.
- Implement authentication and authorization using ASP.NET Core Identity or third-party providers.
- Protect against common vulnerabilities (e.g., SQL Injection, XSS) by validating and sanitizing inputs.
6. Performance Optimization:
- Enable caching strategies (in-memory, distributed) to reduce database load.
- Use asynchronous programming to improve scalability and responsiveness.
- Optimize database queries and use Entity Framework Core’s features like lazy loading and query splitting.
7. Testing:
- Write unit tests for business logic and integration tests for data access and external dependencies.
- Use testing frameworks like xUnit, NUnit, and MSTest.
8. Documentation:
- Maintain comprehensive documentation for APIs using tools like Swagger.
- Document code with XML comments and keep README files up to date.
By adhering to these best practices, developers can create ASP.NET Core applications that are not only efficient and performant but also maintainable and scalable.
Useful Tools and Resources
The official ASP.NET documentation is an invaluable resource for developers of all skill levels. It provides comprehensive information on various aspects of ASP.NET Core, from basic concepts to advanced topics.
Key Features:
- Getting Started Guides: Step-by-step tutorials to help beginners set up their development environment and create their first ASP.NET Core application.
- API Reference: Detailed documentation of the ASP.NET Core API, including classes, methods, properties, and events.
- Conceptual Articles: In-depth articles on key topics such as routing, middleware, dependency injection, and authentication.
- Sample Code: Numerous code samples and snippets to illustrate best practices and common use cases.
- Community and Support: Links to community forums, GitHub repositories, and support channels where developers can ask questions and share knowledge.
Using the Documentation:
- Search Functionality: Use the search bar to quickly find specific topics or API references.
- Version Selector: Choose the appropriate version of ASP.NET Core to view relevant documentation.
- Interactive Tutorials: Follow interactive tutorials that provide hands-on experience with building and deploying ASP.NET Core applications.
Recommended Books, Courses, and Tutorials for Mastering ASP.NET Core
Books:
- "Pro ASP.NET Core 3" by Adam Freeman:
- Comprehensive guide covering all aspects of ASP.NET Core development, including MVC, Web API, Blazor, and more.
- Practical examples and detailed explanations make it suitable for both beginners and experienced developers.
- "ASP.NET Core in Action" by Andrew Lock:
- Focuses on building real-world applications with ASP.NET Core.
- Includes coverage of key concepts like dependency injection, middleware, and Razor Pages.
- "Mastering ASP.NET Core 3.1" by Ricardo Peres:
- Advanced guide for developers looking to deepen their understanding of ASP.NET Core.
- Covers best practices, performance optimization, and complex application development.
Courses:
- Pluralsight:
- "Building Web Applications with ASP.NET Core" by Shawn Wildermuth: A comprehensive course covering the fundamentals and advanced topics of ASP.NET Core development.
- "ASP.NET Core Fundamentals" by Scott Allen: deal for beginners, covering the basics of ASP.NET Core, including MVC, Razor Pages, and Entity Framework Core.
- Udemy:
- "The Complete ASP.NET Core MVC Course" by Mosh Hamedani: Covers ASP.NET Core MVC, dependency injection, Entity Framework Core, and more.
- "ASP.NET Core – A Beginner’s Guide to Become an Expert" by Bhrugen Patel: Step-by-step guide from basic to advanced ASP.NET Core concepts.
Tutorials:
- Microsoft Learn:
- "ASP.NET Core Web Development" Learning Path: Series of modules covering various aspects of ASP.NET Core development, including building web apps, working with data, and deploying applications.
- YouTube Channels:
- Programming with Mosh: High-quality tutorials on ASP.NET Core and other .NET technologies.
- FreeCodeCamp.org: Offers in-depth tutorials on ASP.NET Core, web development, and software engineering.
These resources provide a well-rounded approach to mastering ASP.NET Core, from foundational knowledge to advanced topics, enabling developers to build robust, efficient, and maintainable web applications.
Conclusion
Preparing for ASP.NET interviews requires a solid grasp of core concepts, hands-on practice, and continuous learning to stay updated with the latest advancements. By building and deploying sample applications, engaging with the developer community, and utilizing educational resources, you can enhance your proficiency and confidence. For those seeking the best job opportunities in the tech industry, visit Weekday.work to connect with top employers and find your ideal role.