JavaScript is an indispensable programming language that plays a critical role in web development. It is the backbone of interactive and responsive web applications, enabling developers to create captivating user experiences. JavaScript has become a necessary skill for web developers due to its widespread use in both front-end and back-end development.
As technology continues to advance, staying updated with JavaScript interview questions is crucial for developers who want to propel their careers forward. Javascript Interview questions not only evaluate a candidate's technical proficiency but also their problem-solving skills and adaptability to new challenges. In this Guide, we will explore the significance of JavaScript in web development and emphasize the importance of keeping up-to-date with interview questions to ensure professional growth.
As you gear up to tackle these interviews, consider using Weekday.works to get noticed by companies who value your burgeoning JavaScript skills.
JavaScript Fundamentals
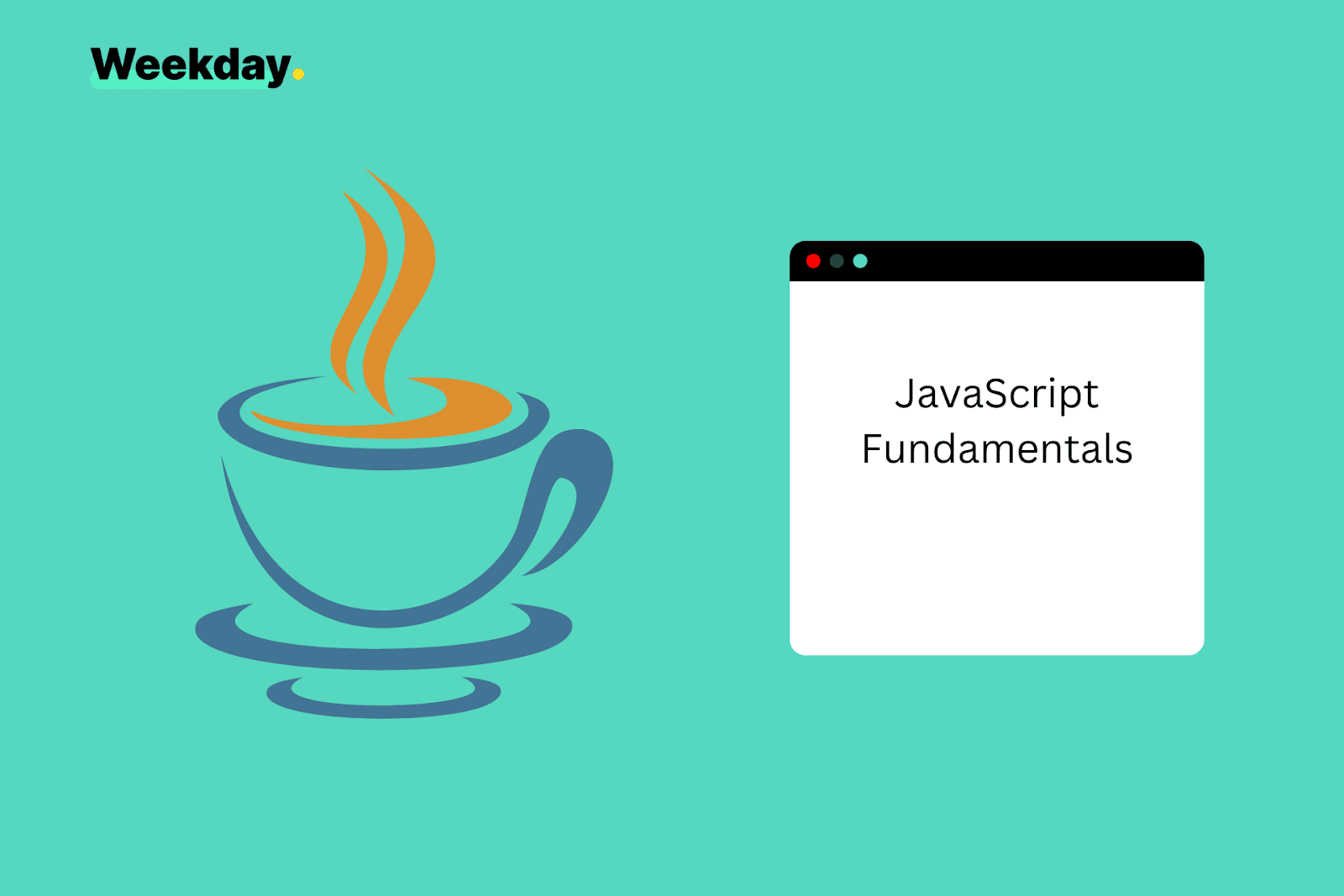
JavaScript is a high-level, interpreted programming language that is widely used in web development. It enables developers to create interactive and dynamic web pages by manipulating the Document Object Model (DOM) and responding to user events. As a high-level language, JavaScript abstracts many of the complexities of computer operations, making it accessible to a broad range of developers. Its interpreted nature means that JavaScript code is executed line by line, allowing for more flexibility and ease of debugging.
Data Types in JavaScript
JavaScript supports several data types to help developers manage various kinds of information:
- Number: Represents both integer and floating-point numbers.
- String: Used for text and characters.
- Boolean: Represents logical values, true or false.
- Null: Indicates the intentional absence of any object value.
- Undefined: Denotes a variable that has been declared but not assigned a value.
- Symbol: Provides unique and immutable values, useful for object property identifiers.
- Object: A collection of properties, where each property is a key-value pair.
- Array: A global object used to construct arrays; a high-level, list-like object.
Hoisting in JavaScript
Hoisting is a unique behavior in JavaScript where variable and function declarations are moved to the top of their containing scope before code execution. This can lead to unexpected results if not properly understood.
Null vs. Undefined
In JavaScript, “null” and “undefined” are two distinct types that represent the absence of a value. Understanding the difference between them is important for writing clear and error-free code.
The “debugger” Keyword
The “debugger” keyword is a powerful tool for debugging JavaScript code. When the debugger statement is encountered, the execution of the code is paused, and the developer can inspect the current state of the program. This allows for a detailed examination of variables, functions, and the call stack, making it easier to identify and fix bugs.
The “this” Keyword
The “this” keyword is a fundamental concept in JavaScript that refers to the context in which a function is executed. It is used to access properties and methods of the object that owns the function. Understanding the behavior of “this” is crucial for writing effective object-oriented code in JavaScript.
Comparing == and ===
Comparing values in JavaScript can be done using either the == operator or the === operator. The == operator performs a value comparison, converting the operands to the same type before comparing them. The === operator, on the other hand, performs a strict comparison, checking both the value and the type of the operands. Understanding the difference between these operators is important for writing accurate and efficient conditional statements.
“var” vs. “let”
The “var” and “let” keywords are used for declaring variables in JavaScript, but they have different scopes. Variables declared with “var” have function scope, meaning they are accessible within the entire function in which they are declared. Variables declared with “let” have block scope, meaning they are only accessible within the block in which they are declared. Understanding the scope of variables is crucial for avoiding unintended side effects and writing cleaner code.
Closures
Closures are a powerful feature in JavaScript that allows functions to access variables from an outer function even after the outer function has completed execution. This concept is fundamental to understanding scope and privacy in JavaScript.
Event Delegation
Event delegation is a technique in JavaScript for handling events efficiently. Instead of attaching event listeners to each child element, a single event listener is attached to a parent element. The event is then delegated to the appropriate child element based on the event target. This reduces the number of event listeners, improving performance and simplifying event management.
Higher-Order Functions in JavaScript
Q: What are higher-order functions in JavaScript?
A: Higher-order functions are functions that can take other functions as arguments or return functions as their results. They are a key feature of functional programming in JavaScript and allow for more abstract and reusable code. An example is the “map()” function, which applies a given function to each element of an array:

Q: What is the purpose of the “bind()” method in JavaScript?
A: The “bind()” method is used to set the “this” value of a function, regardless of how it's called. It creates a new function with a fixed context and any additional arguments passed to the “bind()” method. This is particularly useful for ensuring that callbacks and event handlers have the correct “this” value:
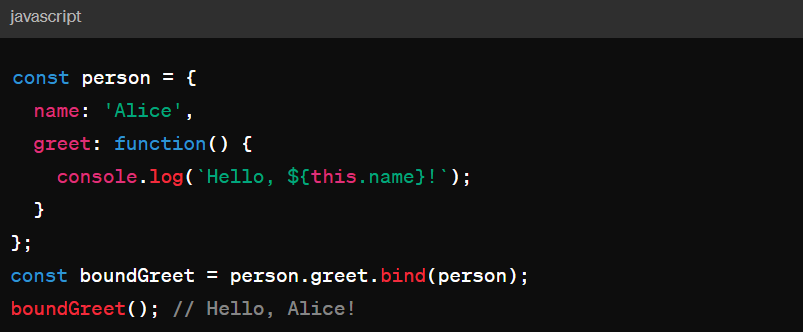
Q: What is the distinction between function declarations and function expressions in JavaScript?
A: Function declarations and expressions are two ways to define functions in JavaScript. A function declaration is hoisted, meaning it can be called before it's defined in the code. A function expression is not hoisted and is typically used when a function needs to be assigned to a variable or passed as an argument to another function:

Q: What are the different types of errors in JavaScript?
A: JavaScript has several types of errors, including:
- Syntax Errors: Occur when the code violates the language's grammar.
- Runtime Errors: Happen during execution, such as trying to access a property of undefined.
- Logical Errors: Mistakes in the logic that lead to incorrect results.
Q: What is memoization in JavaScript?
A: Memoization is an optimization technique that involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. This can significantly improve performance, especially in recursive or computational-heavy functions:
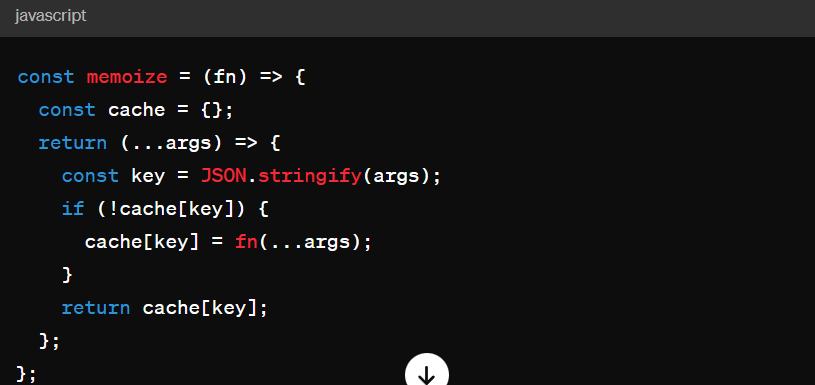
Q: How is recursion used in JavaScript?
A: Recursion is a programming technique where a function calls itself to solve a problem. It's often used for tasks that can be broken down into smaller, similar tasks, such as traversing a tree structure or calculating factorials:
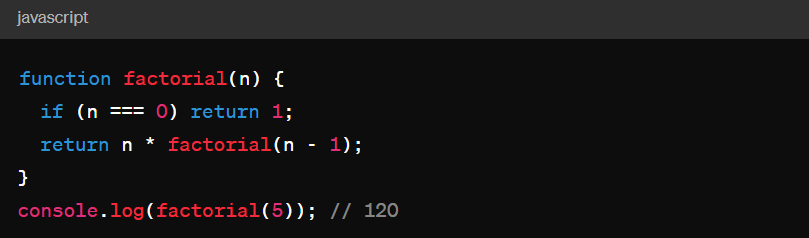
Q: What is the purpose of constructor functions in JavaScript?
A: Constructor functions are used to create objects in JavaScript. They define properties and methods for the objects and are typically called with the new keyword:
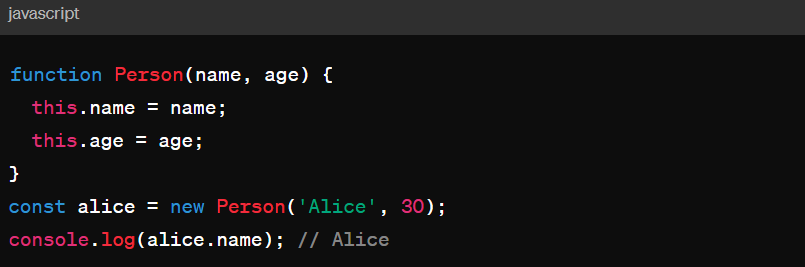
Q: What are callback functions and how are they used in JavaScript?
A: Callback functions are functions passed as arguments to other functions. They are often used in asynchronous operations, event handling, and higher-order functions:

Q: What are Promises and how do they help in handling asynchronous operations in JavaScript?
A: Promises are objects that represent the eventual completion (or failure) of an asynchronous operation. They provide a cleaner and more manageable way to handle asynchronous code compared to callbacks:

Advanced Concepts
Q: What is the role of the “map()” function in JavaScript?
A: The “map()” function in JavaScript is used to apply a function to each element of an array, creating a new array with the results. It is a powerful tool for transforming arrays without modifying the original array:

Q: How do the “splice()” and “slice()” methods differ in JavaScript?
A: The “splice()” method modifies the original array by adding, removing, or replacing elements, whereas the “slice()” method creates a new array with a portion of the original array without altering it:

Q: How does the “reduce()” function simplify arrays to a single value in JavaScript?
A: The “reduce()” function applies a function to each element of an array, accumulating the results into a single value. It is commonly used for tasks such as summing all elements of an array:

Q: What method is used to check if an array includes certain values in JavaScript?
A: The “includes()” method is used to determine if an array includes a certain value, returning true or false as appropriate:

Q: What is the difference between prototype and instance properties in JavaScript?
A: Prototype properties are shared among all instances of a constructor function, whereas instance properties are specific to each instance of an object. Prototype properties are used to define methods and properties that should be inherited by all instances:
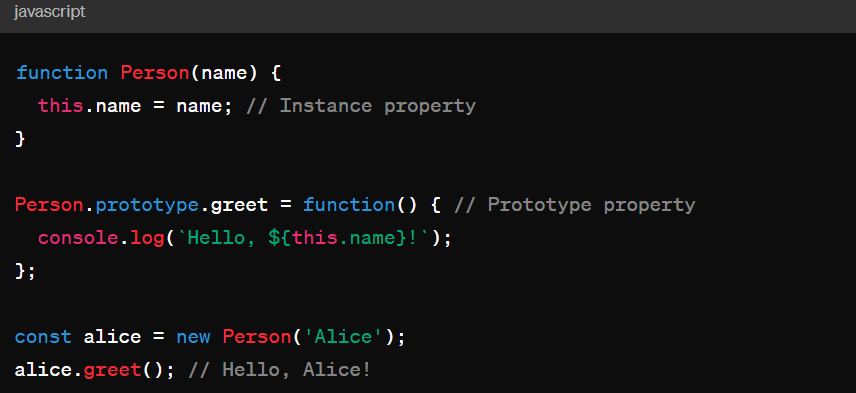
Q: What are the structural differences between an array and an object in JavaScript?
A: Arrays and objects are both used to store collections of data, but they have different structures. Arrays are ordered collections of values, accessed by numeric indices. Objects are collections of key-value pairs, where each key is a string or symbol, and the value can be any type. Arrays are suitable for storing lists of items, while objects are more suited for representing structured data with named properties.
How to Prepare for a JavaScript Interview
Review and Master JavaScript Fundamentals: It's essential to have a solid grasp of JavaScript fundamentals, including variables, data types, loops, conditionals, and functions. This foundational knowledge is crucial for tackling more complex problems and demonstrating proficiency during an interview. A deep understanding of these basics enables you to write efficient and effective code and confidently answer questions related to core JavaScript principles.
Comprehensively Understand Key JavaScript Concepts: A thorough understanding of key JavaScript concepts such as closures, hoisting, scope, event delegation, and prototypal inheritance is vital. These concepts often form the basis of technical javascript interview questions, testing your ability to write sophisticated and optimized code. A comprehensive grasp of these topics allows you to approach problem-solving with an informed perspective, showcasing your expertise and ability to tackle complex challenges.
Study Common Javascript Interview Questions: Familiarizing yourself with common javascript interview questions is a crucial part of your preparation. Practicing your responses to these questions helps you refine your problem-solving skills, improve your understanding of key concepts, and reduce anxiety during the actual interview. This preparation enables you to approach the interview with confidence and effectively demonstrate your knowledge.
Enhance Debugging Skills and Practice: Debugging is an integral part of the development process, and strong debugging skills are highly valued by employers. Regular practice in identifying, analyzing, and fixing errors enhances your problem-solving abilities, attention to detail, and understanding of JavaScript code execution. These skills set you apart in the interview process and demonstrate your competence as a developer.
Importance of Practical Coding Sessions: Practical coding sessions are crucial for applying theoretical knowledge to real-world scenarios. They provide hands-on experience with writing, testing, and optimizing JavaScript code, improving your coding speed, accuracy, and problem-solving abilities. These sessions also prepare you for coding challenges and whiteboard exercises commonly encountered in interviews, building confidence in your coding skills.
Build Projects to Demonstrate Understanding and Application: Building projects is an excellent way to demonstrate your understanding and application of JavaScript. Projects allow you to apply your knowledge in a practical context, showcasing your skills in problem-solving, design, and implementation. They provide tangible evidence of your capabilities to potential employers and can be a focal point in your portfolio.
Participate in Mock Interviews: Participating in mock interviews helps you practice your communication skills, enabling you to articulate your thoughts and solutions clearly and concisely. It also provides an opportunity to receive constructive feedback and refine your responses. Mock interviews simulate the interview environment, reducing anxiety and building confidence. By becoming more comfortable with the interview format and expectations, you can approach the actual interview with poise and assurance.
While mastering JavaScript is key to acing your interviews, finding the right opportunities is equally important. Weekday.works streamlines this process, connecting you with companies looking for your exact skill set. Make sure you're visible to the right employers as you refine your JavaScript expertise.
Final Thoughts
Prepare for your 2024 JavaScript interviews by strengthening your understanding of JavaScript basics, the latest features, and frameworks like React, Vue, or Angular. Practice coding challenges to enhance problem-solving skills. Remember, demonstrating how you approach problems and communicate is as important as technical knowledge. Keep learning, stay updated, and focus on soft skills too. Good luck!